目录
- ResponseEntity HTTP响应类
- Assert 断言
- ObjectUtils 对象操作
- StringUtils 字符串操作
- CollectionUtils 集合工具
- FileCopyUtils 文件工具
- ResourceUtils 资源处理
- StreamUtils 流处理
- ClassUtils 工具类
- BeanUtils 工具类
- ReflectionUtils 工具类
- Base64Utils
- StandardCharests 标准字符集
- SerializationUtils 序列化工具
- HttpStatus 状态码
参考/来源:
ResponseEntity HTTP响应类
简介
ResponseEntity标识整个http相应:状态码、头部信息以及相应体内容。因此我们可以使用其对http响应实现完整配置。
使用
如果需要使用ResponseEntity,必须在请求点返回,通常在spring rest中实现。
- ResponseEntity是通用类型,因此可以使用任意类型作为响应体:
@GetMapping("/hello")
ResponseEntity<String> hello() {
return new ResponseEntity<>("Hello World!", HttpStatus.OK);
}
- 设置http响应头:
@GetMapping("/customHeader")
ResponseEntity<String> customHeader() {
HttpHeaders headers = new HttpHeaders();
headers.add("Custom-Header", "foo");
return new ResponseEntity<>(
"Custom header set", headers, HttpStatus.OK);
}
ResponseEntity提供了两个内嵌的构建器接口: HeadersBuilder 和其子接口 BodyBuilder。
因此我们能通过ResponseEntity的静态方法直接访问。
最简单的情况是相应包括一个主体及http 200响应码:
@GetMapping("/hello") ResponseEntity<String> hello() { return ResponseEntity.ok("Hello World!"); }
大多数常用的http 响应码,可以通过下面static方法:
BodyBuilder accepted(); BodyBuilder badRequest(); BodyBuilder created(java.net.URI location); HeadersBuilder<?> noContent(); HeadersBuilder<?> notFound(); BodyBuilder ok();
另外,可以能使用BodyBuilder status(HttpStatus status)和BodyBuilder status(int status) 方法设置http状态。
使用ResponseEntity BodyBuilder.body(T body)设置http响应体:
@GetMapping("/age") ResponseEntity<String> age(@RequestParam("yearOfBirth") int yearOfBirth) { if (isInFuture(yearOfBirth)) { return ResponseEntity.badRequest() .body("Year of birth cannot be in the future"); } return ResponseEntity.status(HttpStatus.OK) .body("Your age is " + calculateAge(yearOfBirth)); }
也可以自定义头信息:
@GetMapping("/customHeader") ResponseEntity<String> customHeader() { return ResponseEntity.ok() .header("Custom-Header", "foo") .body("Custom header set"); }
因为BodyBuilder.body()返回ResponseEntity 而不是 BodyBuilder,需要最后调用。注意使用HeaderBuilder 不能设置任何响应体属性。
尽管ResponseEntity非常强大,但不应该过度使用。在一些简单情况下,还有其他方法能满足我们的需求,使代码更整洁。
Assert 断言
断言是一个逻辑判断,用于检查不应该发生的情况
// 要求参数 object 必须为非空(Not Null),否则抛出异常,不予放行
// 参数 message 参数用于定制异常信息。
void notNull(Object object, String message)
// 要求参数必须空(Null),否则抛出异常,不予『放行』。
// 和 notNull() 方法断言规则相反
void isNull(Object object, String message)
// 要求参数必须为真(True),否则抛出异常,不予『放行』。
void isTrue(boolean expression, String message)
// 要求参数(List/Set)必须非空(Not Empty),否则抛出异常,不予放行
void notEmpty(Collection collection, String message)
// 要求参数(String)必须有长度(即,Not Empty),否则抛出异常,不予放行
void hasLength(String text, String message)
// 要求参数(String)必须有内容(即,Not Blank),否则抛出异常,不予放行
void hasText(String text, String message)
// 要求参数是指定类型的实例,否则抛出异常,不予放行
void isInstanceOf(Class type, Object obj, String message)
// 要求参数 `subType` 必须是参数 superType 的子类或实现类,否则抛出异常,不予放行
void isAssignable(Class superType, Class subType, String message)
ObjectUtils 对象操作
获取对象的基本信息
// 获取对象的类名。参数为 null 时,返回字符串:"null" String nullSafeClassName(Object obj) // 参数为 null 时,返回 0 int nullSafeHashCode(Object object) // 参数为 null 时,返回字符串:"null" String nullSafeToString(boolean[] array) // 获取对象 HashCode(十六进制形式字符串)。参数为 null 时,返回 0 String getIdentityHexString(Object obj) // 获取对象的类名和 HashCode。参数为 null 时,返回字符串:"" String identityToString(Object obj) // 相当于 toString()方法,但参数为 null 时,返回字符串:"" String getDisplayString(Object obj)
判断工具
// 判断数组是否为空 boolean isEmpty(Object[] array) // 判断参数对象是否是数组 boolean isArray(Object obj) // 判断数组中是否包含指定元素 boolean containsElement(Object[] array, Object element) // 相等,或同为 null时,返回 true boolean nullSafeEquals(Object o1, Object o2) /* 判断参数对象是否为空,判断标准为: Optional: Optional.empty() Array: length == 0 CharSequence: length == 0 Collection: Collection.isEmpty() Map: Map.isEmpty() */ boolean isEmpty(Object obj)
其他工具方法
// 向参数数组的末尾追加新元素,并返回一个新数组 <A, O extends A> A[] addObjectToArray(A[] array, O obj) // 原生基础类型数组 --> 包装类数组 Object[] toObjectArray(Object source)
StringUtils 字符串操作
字符串判断工具
// 判断字符串是否为 null,或 ""。注意,包含空白符的字符串为非空 boolean isEmpty(Object str) // 判断字符串是否是以指定内容结束。忽略大小写 boolean endsWithIgnoreCase(String str, String suffix) // 判断字符串是否已指定内容开头。忽略大小写 boolean startsWithIgnoreCase(String str, String prefix) // 是否包含空白符 boolean containsWhitespace(String str) // 判断字符串非空且长度不为 0,即,Not Empty boolean hasLength(CharSequence str) // 判断字符串是否包含实际内容,即非仅包含空白符,也就是 Not Blank boolean hasText(CharSequence str) // 判断字符串指定索引处是否包含一个子串。 boolean substringMatch(CharSequence str, int index, CharSequence substring) // 计算一个字符串中指定子串的出现次数 int countOccurrencesOf(String str, String sub)
字符串操作工具
// 查找并替换指定子串 String replace(String inString, String oldPattern, String newPattern) // 去除尾部的特定字符 String trimTrailingCharacter(String str, char trailingCharacter) // 去除头部的特定字符 String trimLeadingCharacter(String str, char leadingCharacter) // 去除头部的空白符 String trimLeadingWhitespace(String str) // 去除头部的空白符 String trimTrailingWhitespace(String str) // 去除头部和尾部的空白符 String trimWhitespace(String str) // 删除开头、结尾和中间的空白符 String trimAllWhitespace(String str) // 删除指定子串 String delete(String inString, String pattern) // 删除指定字符(可以是多个) String deleteAny(String inString, String charsToDelete) // 对数组的每一项执行 trim() 方法 String[] trimArrayElements(String[] array) // 将 URL 字符串进行解码 String uriDecode(String source, Charset charset)
路径相关工具方法
// 解析路径字符串,优化其中的 “..” String cleanPath(String path) // 解析路径字符串,解析出文件名部分 String getFilename(String path) // 解析路径字符串,解析出文件后缀名 String getFilenameExtension(String path) // 比较两个两个字符串,判断是否是同一个路径。会自动处理路径中的 “..” boolean pathEquals(String path1, String path2) // 删除文件路径名中的后缀部分 String stripFilenameExtension(String path) // 以 “. 作为分隔符,获取其最后一部分 String unqualify(String qualifiedName) // 以指定字符作为分隔符,获取其最后一部分 String unqualify(String qualifiedName, char separator)
CollectionUtils 集合工具
集合判断工具
// 判断 List/Set 是否为空 boolean isEmpty(Collection<?> collection) // 判断 Map 是否为空 boolean isEmpty(Map<?,?> map) // 判断 List/Set 中是否包含某个对象 boolean containsInstance(Collection<?> collection, Object element) // 以迭代器的方式,判断 List/Set 中是否包含某个对象 boolean contains(Iterator<?> iterator, Object element) // 判断 List/Set 是否包含某些对象中的任意一个 boolean containsAny(Collection<?> source, Collection<?> candidates) // 判断 List/Set 中的每个元素是否唯一。即 List/Set 中不存在重复元素 boolean hasUniqueObject(Collection<?> collection)
集合操作工具
// 将 Array 中的元素都添加到 List/Set 中 <E> void mergeArrayIntoCollection(Object array, Collection<E> collection) // 将 Properties 中的键值对都添加到 Map 中 <K,V> void mergePropertiesIntoMap(Properties props, Map<K,V> map) // 返回 List 中最后一个元素 <T> T lastElement(List<T> list) // 返回 Set 中最后一个元素 <T> T lastElement(Set<T> set) // 返回参数 candidates 中第一个存在于参数 source 中的元素 <E> E findFirstMatch(Collection<?> source, Collection<E> candidates) // 返回 List/Set 中指定类型的元素。 <T> T findValueOfType(Collection<?> collection, Class<T> type) // 返回 List/Set 中指定类型的元素。如果第一种类型未找到,则查找第二种类型,以此类推 Object findValueOfType(Collection<?> collection, Class<?>[] types) // 返回 List/Set 中元素的类型 Class<?> findCommonElementType(Collection<?> collection)
FileCopyUtils 文件处理
输入
// 从文件中读入到字节数组中 byte[] copyToByteArray(File in) // 从输入流中读入到字节数组中 byte[] copyToByteArray(InputStream in) // 从输入流中读入到字符串中 String copyToString(Reader in)
输出
// 从字节数组到文件 void copy(byte[] in, File out) // 从文件到文件 int copy(File in, File out) // 从字节数组到输出流 void copy(byte[] in, OutputStream out) // 从输入流到输出流 int copy(InputStream in, OutputStream out) // 从输入流到输出流 int copy(Reader in, Writer out) // 从字符串到输出流 void copy(String in, Writer out)
ResourceUtils 资源处理
从资源路径获取文件
// 判断字符串是否是一个合法的 URL 字符串。 static boolean isUrl(String resourceLocation) // 获取 URL static URL getURL(String resourceLocation) // 获取文件(在 JAR 包内无法正常使用,需要是一个独立的文件) static File getFile(String resourceLocation)
Resource
// 文件系统资源 D:\... FileSystemResource // URL 资源,如 file://... http://... UrlResource // 类路径下的资源,classpth:... ClassPathResource // Web 容器上下文中的资源(jar 包、war 包) ServletContextResource // 判断资源是否存在 boolean exists() // 从资源中获得 File 对象 File getFile() // 从资源中获得 URI 对象 URI getURI() // 从资源中获得 URI 对象 URL getURL() // 获得资源的 InputStream InputStream getInputStream() // 获得资源的描述信息 String getDescription()
StreamUtils 流处理
输入
void copy(byte[] in, OutputStream out) int copy(InputStream in, OutputStream out) void copy(String in, Charset charset, OutputStream out) long copyRange(InputStream in, OutputStream out, long start, long end)
输出
byte[] copyToByteArray(InputStream in) String copyToString(InputStream in, Charset charset) // 舍弃输入流中的内容 int drain(InputStream in)
ClassUtils 工具类
spring的org.springframework.util
包下的ClassUtils
类,它里面有很多让我们惊喜的功能。
它里面包含了类和对象相关的很多非常实用的方法。
获取对象的所有接口
如果你想获取某个对象的所有接口,可以使用ClassUtils的getAllInterfaces
方法。例如:
Class<?>[] allInterfaces = ClassUtils.getAllInterfaces(new User());
获取某个类的包名
如果你想获取某个类的包名,可以使用ClassUtils的getPackageName
方法。例如:
String packageName = ClassUtils.getPackageName(User.class);
System.out.println(packageName);
判断某个类是否内部类
如果你想判断某个类是否内部类,可以使用ClassUtils的isInnerClass
方法。例如:
System.out.println(ClassUtils.isInnerClass(User.class));
判断对象是否代理对象
如果你想判断对象是否代理对象,可以使用ClassUtils的isCglibProxy
方法。例如:
System.out.println(ClassUtils.isCglibProxy(new User()));
BeanUtils 工具类
spring给我们提供了一个JavaBean
的工具类,它在org.springframework.beans
包下面,它的名字叫做:BeanUtils
。
拷贝对象的属性
曾几何时,你有没有这样的需求:把某个对象中的所有属性,都拷贝到另外一个对象中。这时就能使用BeanUtils的copyProperties
方法。例如:
User user1 = new User();
user1.setId(1L);
user1.setName("苏三说技术");
user1.setAddress("成都");
User user2 = new User();
BeanUtils.copyProperties(user1, user2);
System.out.println(user2);
实例化某个类
如果你想通过反射实例化一个类的对象,可以使用BeanUtils的instantiateClass
方法。例如:
User user = BeanUtils.instantiateClass(User.class);
System.out.println(user);
获取指定类的指定方法
如果你想获取某个类的指定方法,可以使用BeanUtils的findDeclaredMethod
方法。例如:
Method declaredMethod = BeanUtils.findDeclaredMethod(User.class, "getId");
System.out.println(declaredMethod.getName());
获取指定方法的参数
如果你想获取某个方法的参数,可以使用BeanUtils的findPropertyForMethod
方法。例如:
Method declaredMethod = BeanUtils.findDeclaredMethod(User.class, "getId");
PropertyDescriptor propertyForMethod = BeanUtils.findPropertyForMethod(declaredMethod);
System.out.println(propertyForMethod.getName());
ReflectionUtils 工具类
有时候,我们需要在项目中使用反射
功能,如果使用最原始的方法来开发,代码量会非常多,而且很麻烦,它需要处理一大堆异常以及访问权限等问题。
spring给我们提供了一个ReflectionUtils
工具,它在org.springframework.util
包下面。
获取方法
如果你想获取某个类的某个方法,可以使用ReflectionUtils类的findMethod
方法。例如:
Method method = ReflectionUtils.findMethod(User.class, "getId");
获取字段
如果你想获取某个类的某个字段,可以使用ReflectionUtils类的findField
方法。例如:
Field field = ReflectionUtils.findField(User.class, "id");
执行方法
如果你想通过反射调用某个方法,传递参数,可以使用ReflectionUtils类的invokeMethod
方法。例如:
ReflectionUtils.invokeMethod(method, springContextsUtil.getBean(beanName), param);
判断字段是否常量
如果你想判断某个字段是否常量,可以使用ReflectionUtils类的isPublicStaticFinal
方法。例如:
Field field = ReflectionUtils.findField(User.class, "id");
System.out.println(ReflectionUtils.isPublicStaticFinal(field));
判断是否equals方法
如果你想判断某个方法是否equals方法,可以使用ReflectionUtils类的isEqualsMethod
方法。例如:
Method method = ReflectionUtils.findMethod(User.class, "getId");
System.out.println(ReflectionUtils.isEqualsMethod(method));
Base64Utils
有时候,为了安全考虑,需要将参数只用base64
编码。
这时就能直接使用org.springframework.util
包下的Base64Utils
工具类。
它里面包含:encode
和decode
方法,用于对数据进行加密和解密。
String str = "abc";
String encode = new String(Base64Utils.encode(str.getBytes()));
System.out.println("加密后:" + encode);
try {
String decode = new String(Base64Utils.decode(encode.getBytes()), "utf8");
System.out.println("解密后:" + decode);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
执行结果:
加密后:YWJj
解密后:abc
StandardCharsets 标准字符集
我们在做字符转换的时候,经常需要指定字符编码,比如:UTF-8、ISO-8859-1等等。
这时就可以直接使用java.nio.charset
包下的StandardCharsets
类中静态变量。
例如:
String str = "abc";
String encode = new String(Base64Utils.encode(str.getBytes()));
System.out.println("加密后:" + encode);
String decode = new String(Base64Utils.decode(encode.getBytes())
, StandardCharsets.UTF_8);
System.out.println("解密后:" + decode);
SerializationUtils 序列化工具
有时候,我们需要把数据进行序列化
和反序列化
处理。
传统的做法是某个类实现Serializable
接口,然后重新它的writeObject
和readObject
方法。
但如果使用org.springframework.util
包下的SerializationUtils
工具类,能更轻松实现序列化和反序列化功能。例如:
Map<String, String> map = Maps.newHashMap();
map.put("a", "1");
map.put("b", "2");
map.put("c", "3");
byte[] serialize = SerializationUtils.serialize(map);
Object deserialize = SerializationUtils.deserialize(serialize);
System.out.println(deserialize);
HttpStatus 状态码
很多时候,我们会在代码中定义http的返回码,比如:接口正常返回200,异常返回500,接口找不到返回404,接口不可用返回502等。
private int SUCCESS_CODE = 200;
private int ERROR_CODE = 500;
private int NOT_FOUND_CODE = 404;
其实org.springframework.http
包下的HttpStatus枚举,或者org.apache.http
包下的HttpStatus
接口,已经把常用的http返回码给我们定义好了,直接拿来用就可以了,真的不用再重复定义了。
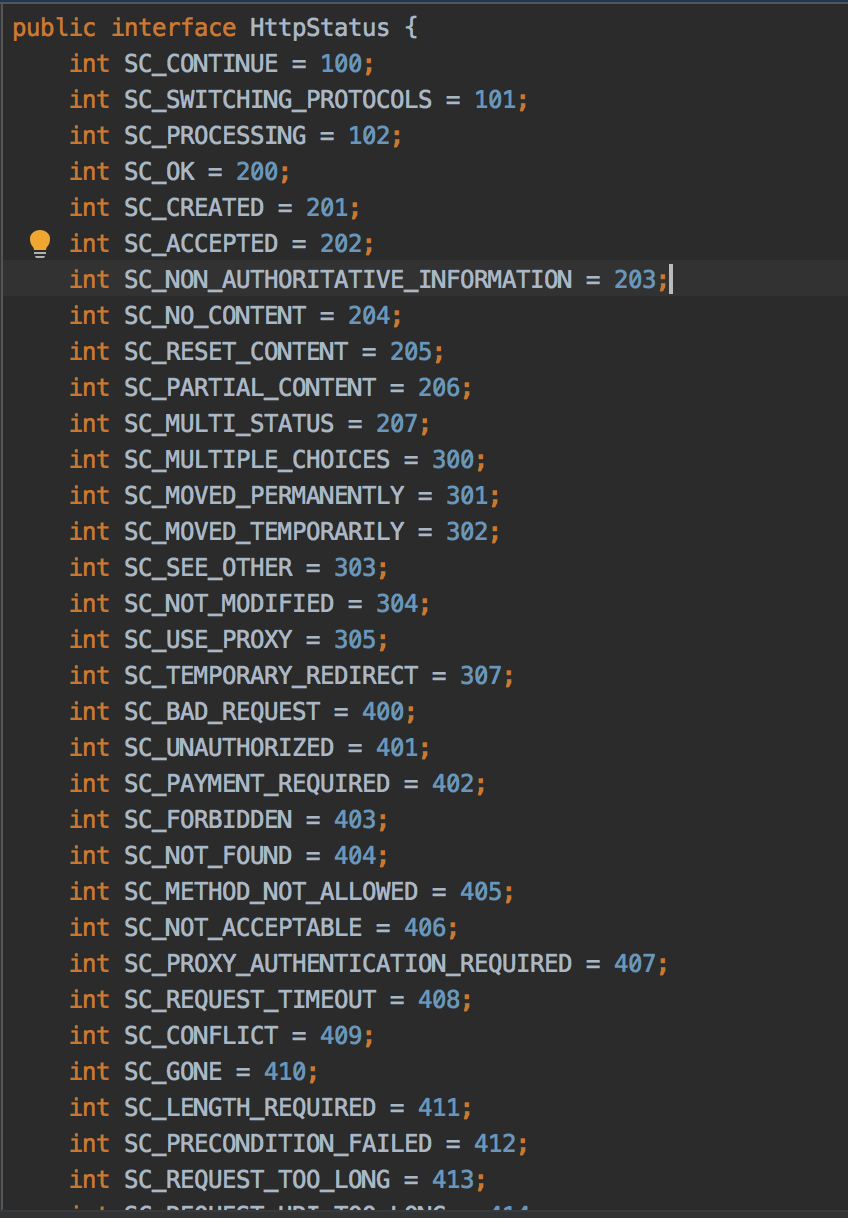